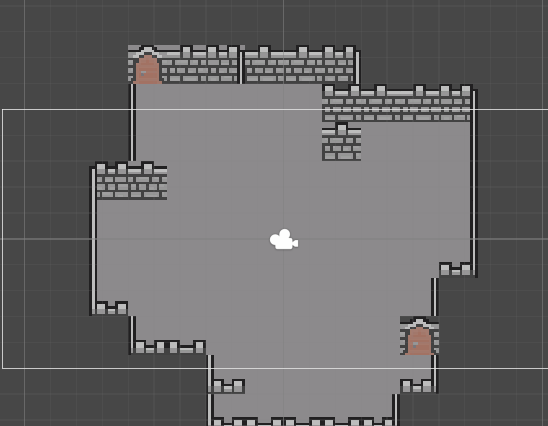
Creating random terrains and 2D levels involves various algorithms. In this post, I'll explore using a Random Walk generation approach.
A random walk generation algorithm is a mathematical formula that determines the next location for element placement. Imagine a walker moving on a grid, taking random steps (up, down, left, or right) at each turn. This algorithm replicates that movement to create a path or fill an area.
Here's a simplified example of the random walk equation in two dimensions (X and Y):
(Xn+1, Yn+1) = (Xn, Yn) + (ϵx, ϵy)
Let's break down the equation:
With each step, the walker moves to the new calculated square and repeats the process, generating a random path.
To ensure the walker doesn't occupy the same space twice, we can check if a square is already filled before placing something there. If it's empty, we proceed with generation.
Assigning textures to the tiles involved examining the surrounding tiles of the initial tile. Based on this information, we generated a code that represented the tile's configuration. This code was then matched against a database to determine the appropriate texture.
The generated code might look something like this, depending on the tile's position relative to its neighbors:
-0b1111 (all sides connected) -0b10100000 (top and bottom connected) -0b10000110 (right and bottom connected) -0b0101 (top and left connected)
This code is then used to set the tile's texture in the tilemap.
if (code == wallFullCode) {
tile = wallFull;
}
(Note: This is a simplified representation. The actual code might involve a lookup table or a more complex logic depending on your implementation.)
Random walk generation is a useful technique for creating interesting and varied dungeon terrains. If you have any further questions, feel free to check out my YouTube channel, where I'll be uploading a devlog on this topic soon!
YouTube ChannelThank you for reading!